Updating a database connection string across multiple servers means changing the configuration file on each machine. If even one server is missed or an incorrect value is entered, some applications will continue using outdated settings, leading to unexpected connection failures and downtime. The same issue occurs with firewall rules, logging settings, and security policies. If servers use different versions of a configuration file, some may allow unauthorized access, while others enforce stricter rules, creating inconsistencies that are difficult to track and troubleshoot.
Terraform template files (.tpl or .tftpl) solve this problem by automating the creation of configuration files after instances are deployed. Instead of maintaining multiple hardcoded files for different servers, a single template file is created with placeholders for values like database credentials, backend addresses, firewall rules, and authentication settings. Terraform processes the template and replaces these placeholders with actual values based on the current infrastructure state, ensuring that every instance gets the correct configuration.
What are Terraform Template Files
Template files are used to generate configuration files, service definitions, and initialization scripts that set up instances and services at deployment. Unlike .tf files, which define cloud resources like EC2 instances, VPCs, and IAM roles, template files create structured configuration files that control how instances and applications operate after provisioning.
For example, when a new server is deployed, an initialization script generated from a template can install required software, configure system settings, and apply security policies. This script might install Nginx, Docker, or PostgreSQL, adjust kernel parameters for performance tuning, enable firewall rules to restrict access, or set SSH policies to enforce secure authentication. Without templates, these configurations would need to be written manually for each server, increasing the risk of inconsistencies.
Beyond instance setup, Terraform templates are commonly used to generate service configuration files. Instead of writing and maintaining separate files for each server, a template file can generate them with the correct values at deployment.
For example, an Nginx configuration file defines how the server handles incoming requests. It specifies which domains the server should respond to, which backend servers should receive traffic, and whether SSL should be enabled. A database configuration file includes the database hostname, port number, username, and password, ensuring applications always connect using the correct credentials. A logging configuration file defines where logs should be stored, how often they should be rotated, and which types of logs (errors, warnings, access logs) should be collected.
Terraform processes these template files using the templatefile() function, replacing placeholders with actual values pulled from Terraform variables or resource attributes. These values could be the private IP of an EC2 instance, the hostname of a database server, the port number of a web application, or an API key for authentication. Instead of manually updating multiple configuration files, a single template file ensures that every instance is set up correctly, preventing misconfigurations and simplifying deployments.
Terraform Templates in Infrastructure Automation
We use Terraform templates to dynamically generate configuration files, eliminating the need for manually maintaining and updating static files such as cloud-init scripts, application configuration files, and infrastructure provisioning scripts. Configuration management in infrastructure typically involves defining operating system settings, network configurations, service parameters, package installations, and user permissions for virtual machines, containers, and cloud resources.
Automation of resource config
Instead of maintaining multiple static configuration files such as .config or .env for different instances or services, Terraform template files .tftpl allow these files to be generated at deployment based on real-time infrastructure data such as EC2 instance private IP addresses database hostnames. For a better understanding, let's go through an example that demonstrates how templates play an important role in our deployments.
Let's say we need to configure an EC2 instance with a custom hostname and install the necessary packages at startup. So, instead of maintaining separate scripts for each instance, we can create a Terraform template file that defines the required setup and allows .tftpl file to generate this configuration post-deployment. The template file, cloud-init.tftpl
, contains:
The template file cloud-init.tftpl
follows the cloud-init format, which allows automated instance initialization. The #cloud-config
directive at the start of the file ensures that the instance recognizes the configuration and applies it during boot.
This template includes a placeholder ${hostname}
that Terraform replaces with the actual hostname for each instance. We define the hostname in Terraform using a variable:
Then, we use Terraform’s templatefile()
function to read the template and inject the hostname dynamically:
Terraform processes the template, replaces ${hostname}
with the value from var.server_name
, and applies the final configuration to the instance’s user_data
.
Now, after connecting the deployed ec2 instance using ssh -i my-key.pem ec2-user@13.61.187.128
, we can see

To verify nginx installation, we run nginx -v
, which returns:-

This ensures consistency across different environments while eliminating the need for redundant scripts. Let's move on to how these templates should be structured for clarity.
Structuring Terraform Template Files
Organizing Terraform template files properly ensures that configurations remain easy to manage, understand, and modify. A structured approach prevents confusion when working with multiple templates and reduces the risk of applying incorrect configurations. Using .tftpl as the standard extension instead of .tpl makes it clear that the file is intended for Terraform processing, distinguishing it from other generic template files that might be used in different applications.
Naming template files descriptively helps identify their purpose without needing to inspect their content. For example, a template file named instance-user-data.tftpl explicitly indicates that it generates user-data scripts for instances, while a file named nginx-config.tftpl suggests it is responsible for generating an Nginx configuration. In contrast, using generic names like config.tftpl or template.tftpl provides little context, making it harder to identify the file’s purpose when managing multiple templates in a project.
Beyond naming conventions, structuring Terraform templates effectively also involves defining placeholders for variables in a way that keeps the file readable. Instead of hardcoding values like database credentials or hostnames directly into templates, variables are referenced using ${variable_name}, ensuring that Terraform replaces them with actual values at deployment. This approach removes the need for maintaining separate template files for different environments, as a single template can generate the correct configuration based on provided input values.
For example, instead of creating separate Nginx configuration templates for different environments, a single nginx-config.tftpl
file can contain placeholders for server names, backend addresses, and SSL settings, allowing Terraform to populate them dynamically. This makes template files reusable, easier to update, and scalable for managing infrastructure configurations efficiently.
With a well-structured approach, Terraform templates eliminate duplication, enforce consistency across deployments, and ensure that infrastructure settings are applied correctly every time. Now, let’s explore how variables can be incorporated within Terraform templates to further enhance flexibility and maintainability.
Using Variables in Templates
Terraform template files allow configurations to be generated with real-time deployment values, eliminating the need for hardcoded configs. Variables in templates ensure that parameters such as hostnames, database credentials, service ports, and API endpoints are set dynamically when Terraform applies the configuration. This prevents the need to maintain separate versions of configuration files for different deployments.
Inside a .tftpl file, variables are referenced using the ${variable_name}
notation. When Terraform processes the template, it replaces these placeholders with actual values pulled from Terraform variables or resource outputs. For example, let's say we need to configure an EC2 instance and customize its cloud-init script with different hostnames depending on the environment. Instead of writing separate configuration files for each case, we can define a reusable template file cloud-init.tftpl like this:
In Terraform, we can then pass values to this template using the templatefile() function:
Here, Terraform reads the cloud-init.tftpl file, replaces the ${hostname}
placeholder with the value provided in var.server_name
, and applies the final configuration when launching the EC2 instance. This method ensures that configurations remain flexible and reusable across different deployments, minimizing redundant files and manual updates in config files. Now, let’s explore how we can add logical conditions or loops in our template files.
Adding Logic to Templates: Conditionals and Loops
Terraform templates provide built-in logic handling capabilities, including conditionals and loops, making them more adaptable for various infrastructure requirements. Conditionals allow specific sections of a configuration to be included or excluded based on predefined variables. For instance, when enabling logging for an application, a conditional block can be used:
If enable_logging
is set to true, this block will be included in the final configuration; otherwise, it will be omitted.
Loops within Terraform templates make it possible to iterate over a list of values, generating multiple configuration entries without writing redundant lines. Consider a scenario where we need to configure multiple SSH host entries dynamically. Instead of defining each entry separately, we can use a loop to generate them:
Here, hostnames
is a list variable, and Terraform will iterate over it to create individual Host entries for each value in the list. This is especially useful for generating configurations such as firewall rules, DNS records, or load balancer backends, where multiple entries follow the same format.
These logic constructs significantly reduce the need for separate configuration files while ensuring infrastructure remains flexible and scalable. With conditionals and loops in place, Terraform templates can be customized efficiently to handle different environments and deployment scenarios without excessive manual modifications.
Using Terraform Templates: Hands-On
Using the templatefile() Function
First, we create a user-data.tftpl template for configuring an EC2 instance. This template is responsible for executing commands at startup, allowing us to inject custom configurations into the instance during provisioning.
The template file user-data.tftpl contains the following script:
This script prints a startup message and a personalized welcome message using the ${username}
placeholder, which Terraform will replace with the actual value during deployment.
Next, we define the main.tf file to create the EC2 instance and pass the user-data script.
Terraform processes user-data.tftpl, replaces the ${username}
placeholder with the value from var.username
, and applies it as user_data to the EC2 instance.
After running terraform apply
, Terraform provisions the instance and outputs its public IP.
To connect to our instance, we use SSH:
Upon login, the system displays the default Amazon Linux 2 message along with security update recommendations. To verify that the user-data script executed correctly, we check the cloud-init logs:
The output confirms that the script ran successfully:

"Welcome, User!" in the logs confirms that Terraform successfully processed the template and passed the correct value for ${username}
.
This approach ensures that instance-specific configurations are applied consistently without manually modifying scripts for each deployment.
Using template_file Data Source
Now, first, we define an app-config.tftpl template for application settings. Instead of manually maintaining separate configuration files for different environments, this template allows Terraform to generate a structured configuration file dynamically based on provided values.
This template ensures that the application settings remain consistent across different deployments while allowing flexibility in defining values.
Next, we declare variables in variables.tf to hold the application name, environment, and database connection URL:
These variables allow customization without modifying the template file directly.
The actual values are set in terraform.tfvars:
Now, in main.tf, Terraform reads the template file and replaces the placeholders with values from the variables:
Here, Terraform loads the app-config.tftpl template, replaces variables using data.template_file
and writes the final output to a local file app-config.txt.
Now, after initializing Terraform and applying the configuration.

We can now verify the generated application configuration by:
The output confirms that the template was processed correctly:

This method eliminates the need for manually creating environment-specific configuration files. Simply update terraform.tfvars, run terraform apply
, and Terraform generates the appropriate configuration file automatically.
Implementing Conditionals and Loops in Templates
When managing SSH configurations, manually creating a ssh_config file for one or two servers might seem manageable. However, as the number of servers grows to hundreds, maintaining individual SSH host entries becomes impractical. Instead of manually writing each entry, Terraform template files can automate this process using a loop to dynamically generate SSH host configurations for multiple machines.
Below is a hosts.tftpl
template file that uses a loop to generate SSH host entries for multiple servers:
This template ensures that each server entry is formatted correctly, reducing manual work and avoiding errors when managing multiple SSH connections.
Next, define a variable to hold the list of SSH hosts in variables.tf:
Each host in the list has three properties: name (SSH alias), ip (server IP address), and user (username for login).
The actual values for these hosts are provided in terraform.tfvars:
Now in main.tf
, Terraform reads hosts.tftpl
, processes the variables, and writes the final output to a local file:
This ensures that the ssh_config
file is generated automatically based on the values provided in terraform.tfvars
.
After initialising Terraform and applying the configuration using terraform init && terraform apply, we get
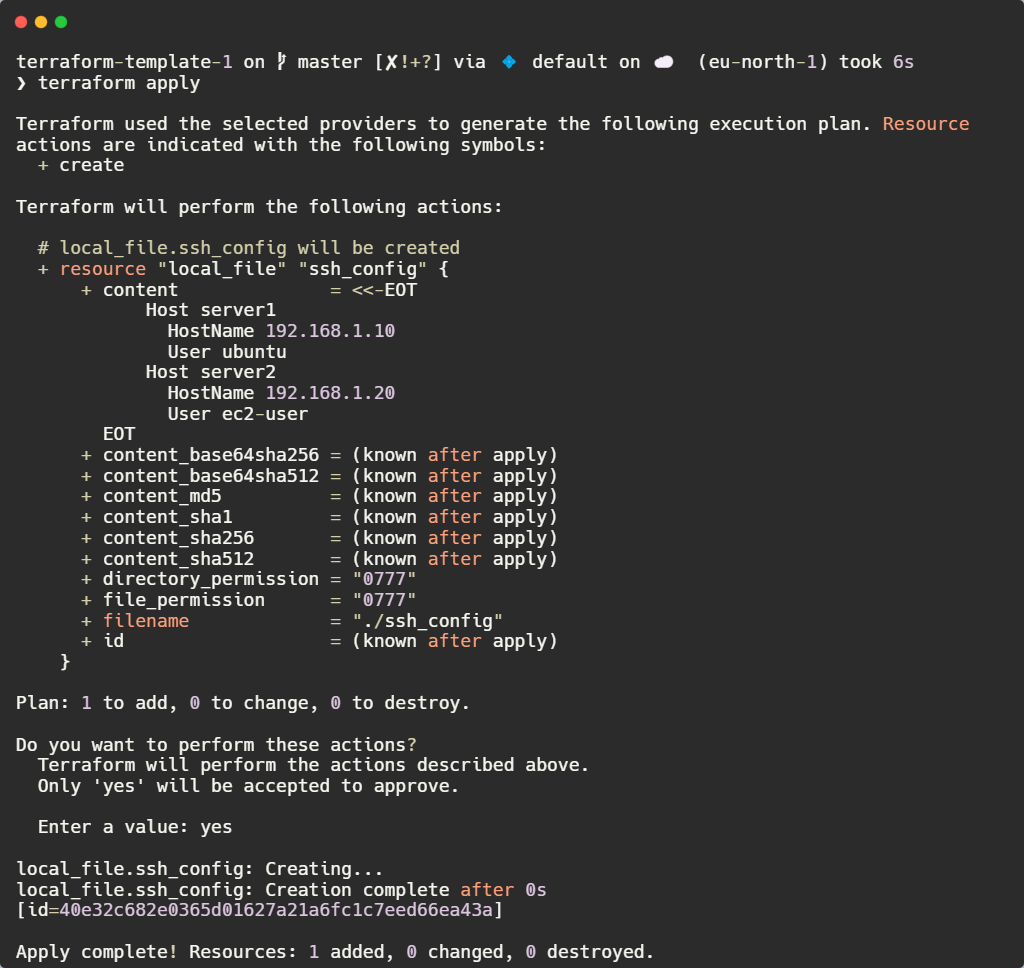
Terraform generates an ssh_config file. To verify its contents, we can run:
To get output that confirms that Terraform correctly formatted the entries:

This approach eliminates the need to manually update the ssh_config
file when adding or modifying servers. Simply update terraform.tfvars
, run terraform apply
, and the configuration is regenerated automatically.
Best Practices for Terraform Templates
Use templatefile() instead of template_file
Older versions of Terraform relied on template_file as a data source to process templates. However, this approach is now outdated. The recommended method is templatefile(), which is built directly into Terraform and does not require additional configuration. This makes template processing faster and eliminates unnecessary dependencies.
Keep templates simple
Templates should only handle text replacement, not complex conditionals or transformations. If advanced logic is needed, it should be managed within Terraform using variables or modules before passing values to the template. This keeps the templates easy to read and avoids unnecessary complexity. When templates contain too much logic, troubleshooting becomes harder, and maintenance becomes a challenge. Instead, let Terraform handle logic separately and only pass finalized values to the template.
Store templates in the version control
Terraform templates should always be stored in a version control system like Git. This ensures that every change is tracked, allowing teams to collaborate without overwriting each other’s modifications. Storing templates alongside Terraform configurations also makes it easier to maintain infrastructure definitions in a structured manner. Keeping templates versioned helps with rollback in case of errors and ensures consistency across deployments.
Validate generated files
Before deploying infrastructure, it's important to verify that the template is producing the correct output. Mistakes in templates, such as missing variables or incorrect formatting, can cause deployment failures. After running Terraform, the generated file should be inspected manually to confirm that it contains the expected values. Testing the output in a staging environment before applying it to production prevents misconfigurations and downtime.
Now, even with Terraform templates, managing your cloud infrastructure at scale can become difficult. When multiple instances and resources are deployed, tracking their status, configuration, and compliance manually is not efficient. You might not know if everything is working correctly or if there are issues like misconfigurations, security risks, or unmanaged resources that aren't being tracked by Terraform.
Firefly Gives Full Visibility into Your Terraform-Managed Infrastructure
Firefly provides a view of your cloud environment, helping teams identify and fix issues faster.
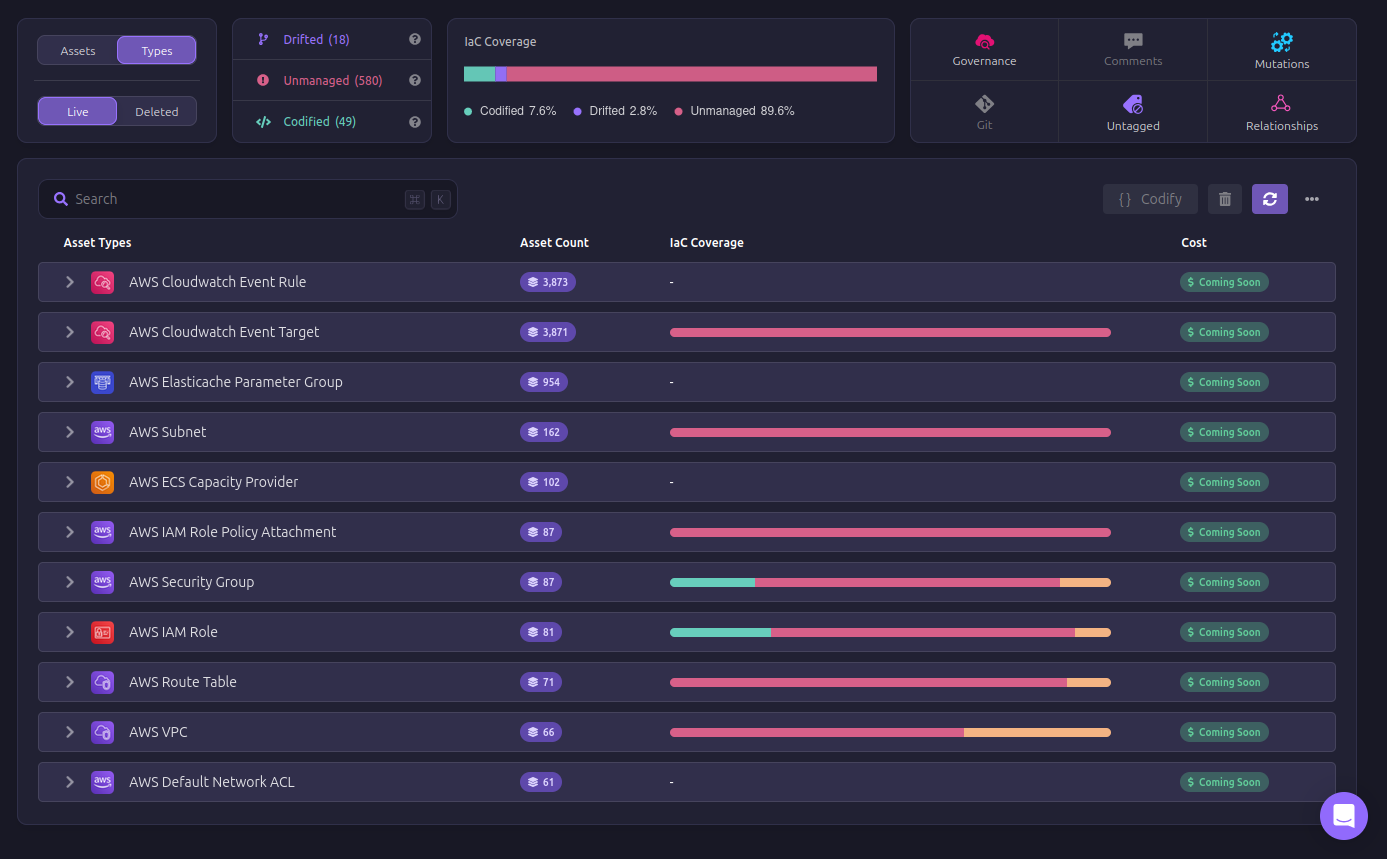
Without this kind of visibility within your resources, teams might struggle to know which resources are properly configured and which ones have drifted away from the intended state. Firefly helps codify these unmanaged resources, bringing them under Terraform’s control to make sure that every component in your infrastructure is properly tracked.

Beyond tracking, Firefly makes governance and compliance easier. The above image shows the governance dashboard, where Firefly automatically detects misconfigurations across networking, encryption, and storage. Instead of manually reviewing each resource for security risks, Firefly automates security checks and suggests fixes. This helps maintain best practices without teams having to spend hours sifting through configuration files.
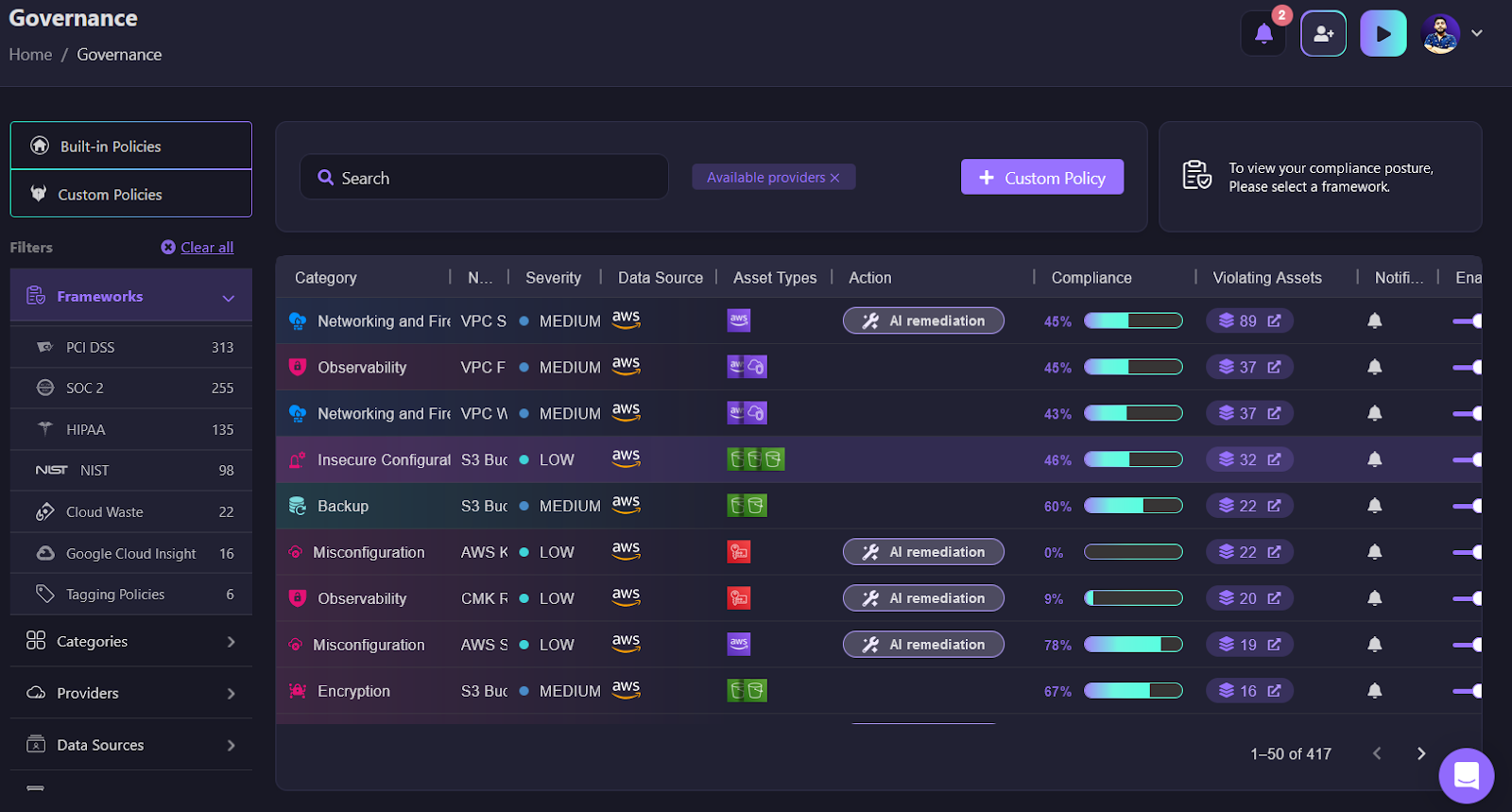
When multiple instances and resources are deployed, identifying which ones are working correctly and which ones need attention becomes difficult. Firefly simplifies this process by giving teams a complete picture of their infrastructure, allowing them to catch and fix issues before they cause downtime or security vulnerabilities.
Conclusion
Till now, you should have a clear understanding of how Terraform templates automate configuration management by dynamically generating files. Instead of maintaining multiple static configurations, the templatefile()
function ensures each instance gets the correct settings using real-time Terraform variables.
FAQs
What is the difference between templatefile() and template_file?
The templatefile()
function is the preferred approach in Terraform versions 0.12 and above. The template_file
data source is now deprecated and should not be used in new configurations.
Can I use loops inside Terraform template files?
Yes, Terraform template files support loops using %{ for item in list } syntax, allowing multiple entries to be generated from a list.

How do I handle optional content in Terraform templates?
Use %{ if condition } blocks to conditionally include or exclude sections based on variable values.
What is the recommended file extension for Terraform template files?
Using .tftpl helps distinguish Terraform templates from other template formats, making them easier to manage.
Can Terraform templates be stored in a remote repository?
Yes, storing templates in a version-controlled repository like Git ensures tracking and collaboration across teams.