What is GitOps Workflow?
GitOps is about managing infrastructure through version-controlled workflows, similar to how developers manage application code. Instead of manually configuring instances or relying on custom scripts, all infrastructure changes are made through pull requests in Git.
When a developer needs to update the infrastructure, like creating a new EC2 instance, they should create a pull request with the necessary Terraform configuration changes. This makes sure that changes are not pushed directly to the main branch, which could lead to unreviewed or potentially risky modifications being deployed to the infrastructure. The pull request is ideally reviewed by team members to make sure that all best practices are followed. Once approved and merged into the main branch, automation tools like GitHub Actions or ArgoCD detect the change and automatically apply it to the cloud infrastructure.
How Git Manages Infrastructure in GitOps
Git isn’t just for version control in GitOps; it’s the central hub for managing infrastructure. Let’s say a DevOps team is managing a Kubernetes cluster across different cloud providers like AWS and GCP. Instead of logging into each cloud console to update resources like node pools or network configurations, they push changes to a Git repository. A CI/CD pipeline, like GitHub Actions or ArgoCD, detects these changes and deploys them automatically. If something goes wrong, like a network rule causing an application to stop working, the team can easily find the issue in the commit history and fix it by rolling back to a previous version using a simple Git revert command.
Declarative vs. Imperative Approaches
Infrastructure management has traditionally relied on imperative workflows, where DevOps teams use scripts to provision their cloud resources. These scripts, often written in Bash, Python, or Ansible, explicitly define how to create infrastructure components like VPCs, subnets, and EC2 instances. While this approach provides control over execution, it also introduces complexity as your environments scale. Teams must make sure that all the configurations are applied in the correct order.
GitOps shifts to a declarative model, where infrastructure is defined in code that specifies the final desired state rather than outlining the exact steps to achieve it. Instead of running scripts to provision instances, a GitOps workflow describes how the infrastructure should look, and automation tools handle the provisioning.
Tools like ArgoCD, Flux, and Terraform Cloud monitor the infrastructure state to make sure that it remains consistent with what is defined in Git. If someone manually modifies an EC2 instance’s configuration through the cloud provider’s console, these tools detect the drift, the mismatch between the actual and declared state, and notify the team. Unlike imperative workflows, where teams must manually correct misconfigurations, declarative GitOps workflows provide a single source of truth and enforce consistency through automated reconciliation.
Core Principles of GitOps
Now, GitOps isn’t just about automation - it’s about making sure your infrastructure is consistent, secure, and efficient when working at scale. Let’s break down the core principles that make GitOps effective:
Declarative Infrastructure
GitOps follows a declarative approach, meaning the desired state of infrastructure is defined in code. Instead of manually provisioning resources, teams describe their infrastructure configuration, and GitOps tools make sure that the cloud environment reflects that definition as well. This eliminates the risk of configuration drift caused by ad-hoc manual changes.
For example, if an S3 bucket is required, instead of writing a script that lists every action needed to create it, the infrastructure is described as a configuration file, stating what the final state should be.
Version Control as the Source of Truth
All infrastructure configurations are stored in Git repositories, providing:
- Traceability: Every infrastructure change is recorded in the commit history, making it easy to audit who made changes and why.
- Collaboration: Teams can use pull requests and code reviews to ensure infrastructure updates follow best practices.
- Rollback Capabilities: If a change introduces issues, reverting to a previous state is as simple as rolling back to an earlier commit.
Git serves as the single source of truth, meaning the infrastructure defined in repositories is always considered the correct state. This prevents inconsistencies between environments and allows for better compliance and security.
Automated Deployment Pipelines
With GitOps, deployments are triggered automatically when changes are made in Git. Instead of relying on interventions from your team’s end, tools like GitHub Actions, ArgoCD, and Terraform Cloud make sure that updates are consistently applied. This process reduces the chances of error and also speeds up the deployment cycles.
For example, when a developer pushes a Terraform configuration change to the main branch, a CI/CD pipeline can be triggered to:
- Validate the Terraform configuration.
- Plan the proposed changes and display the expected modifications.
- Apply the changes to the infrastructure after approval.
This automation makes sure that infrastructure updates are thoroughly tested and reviewed before reaching the production environment.
Continuous Reconciliation
GitOps enforces continuous reconciliation, where tools constantly monitor the actual infrastructure state and compare it against what is defined in Git. If any discrepancies are detected, GitOps tools notify the team or even restore the infrastructure to its correct state.
For example, if a security group rule is modified through the AWS console, GitOps tools will detect the drift and prompt a remediation action, either automatically correcting it or alerting the team to investigate. This ensures that environments remain secure and aligned with defined policies at all times.
By following these principles, GitOps creates a structured, repeatable, and scalable approach to managing your infrastructure. It eliminates configuration drift, reduces deployment risks, and makes sure that teams operate with a consistent infrastructure strategy.
How GitOps Works (Technical Flow)
Now that we understand the core principles of GitOps, let’s now take a look at the technical workflow that makes it this effective. A GitOps pipeline follows a structured flow to ensure infrastructure changes are tracked, validated, and applied correctly.

Change Management Workflow
Every infrastructure update in a GitOps workflow starts with a change request in Git. When a developer needs to modify infrastructure, they create a pull request containing the necessary Terraform configuration changes. The pull request undergoes a review process where team members validate the configuration against best practices and compliance rules before merging it into the main branch.
Once the pull request is merged, automation tools such as GitHub Actions, ArgoCD, or Terraform Cloud detect the change and initiate a deployment process. These tools ensure the infrastructure updates are applied in a controlled, repeatable manner.
Reconciliation Loop
GitOps follows a continuous reconciliation approach to maintain consistency between the declared and actual infrastructure states. Tools like ArgoCD and Flux periodically check the infrastructure state in the prod against the desired state defined in Git. If any discrepancies are found, such as a manually modified security group rule or an unauthorized instance change, the tool flags the drift and initiates corrective actions based on predefined policies.
Feedback Mechanism
An important component of GitOps is real-time feedback on deployments. Monitoring tools integrate with GitOps workflows to provide visibility into changes and infrastructure health. Logs and alerts from Terraform Cloud, Prometheus, or Grafana help teams understand the impact of deployments and troubleshoot issues much more easily.
By structuring infrastructure management around GitOps workflows, teams can make sure that every change is traceable, infrastructure remains consistent, and deployments are executed with minimal risk.
Hands-On: Implementing GitOps for Infrastructure Using Terraform
Now, moving into the hands-on section, we'll go through the implementation of a GitOps workflow using Terraform, GitHub Actions, and AWS. This process involves setting up version control, defining infrastructure as code, automating deployments, and ensuring reconciliation between Git and the live cloud environment.
To implement GitOps using Terraform, we start by setting up a Git repository that will store our infrastructure configuration. This repository will serve as the single source of truth, ensuring all infrastructure changes are tracked and version-controlled.
First, we initialize a new Git repository and push it to GitHub:

Once the repository is set up, we create a directory to hold our Terraform configuration files:
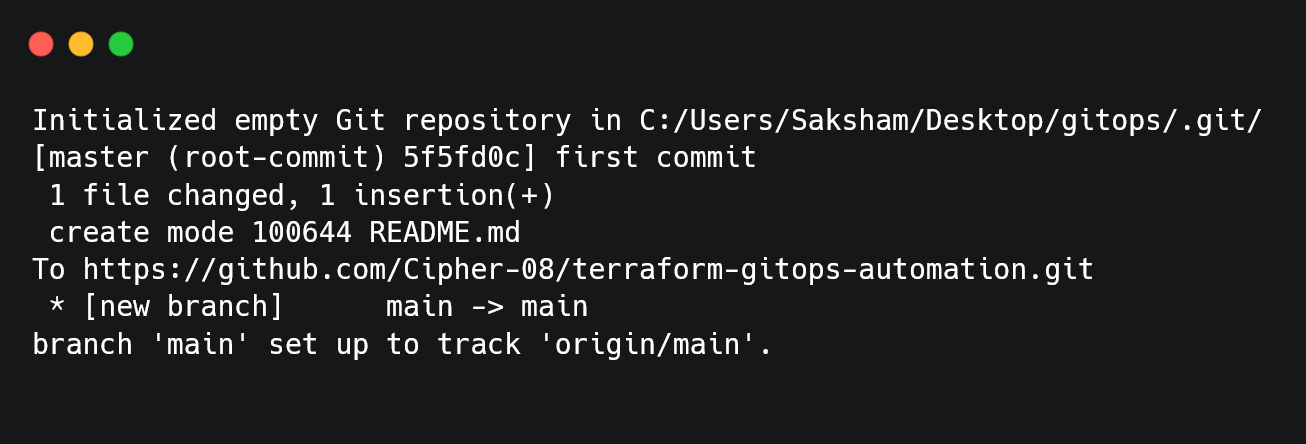
Terraform needs a backend to store the state file, which keeps track of infrastructure resources. We configure an S3 bucket as the backend by adding the following to backend.tf:
In main.tf, we define an S3 bucket as an example infrastructure resource:
This configuration specifies that an S3 bucket named gitops-bucket
should be created in AWS.
Now, to automate infrastructure provisioning, we set up a GitHub Actions workflow. This workflow ensures that whenever a change is pushed to the main branch, Terraform will automatically apply the changes.
We create the required directories:
Then, we define the workflow in .github/workflows/terraform-gitops-workflow.yml:
Finally, we add and push all the changes:
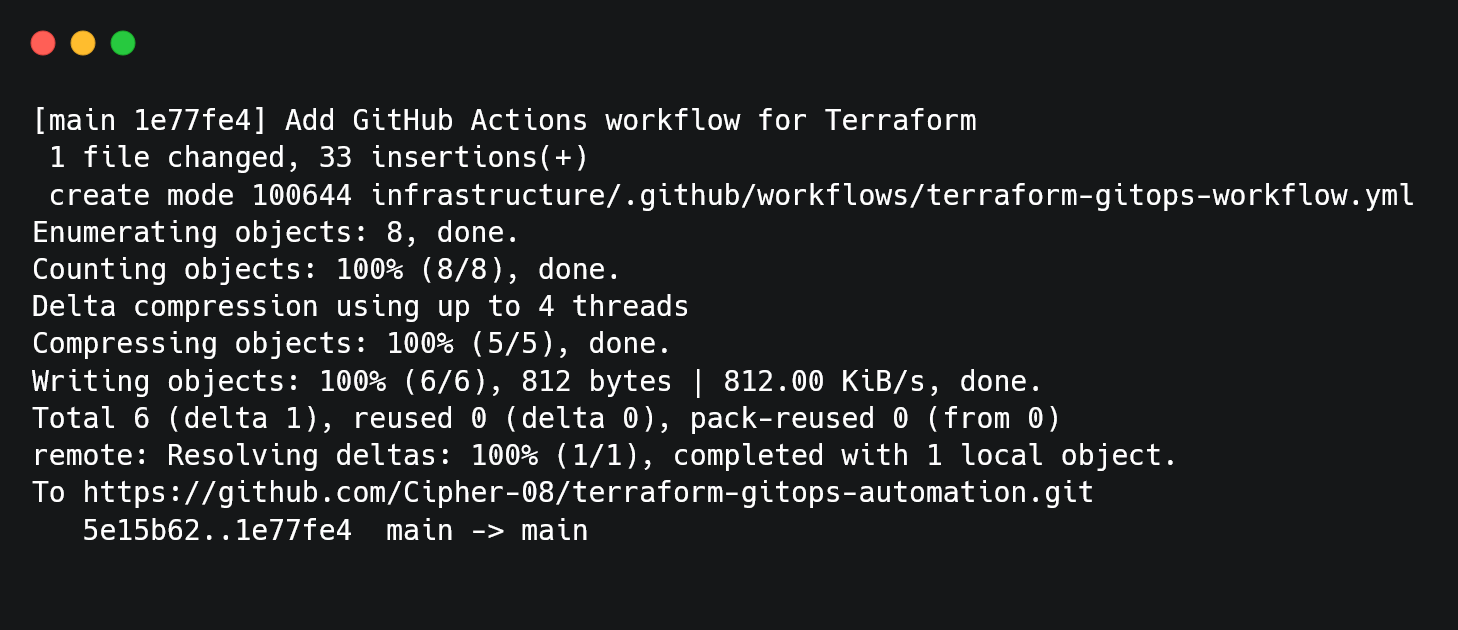
Once the GitHub Actions workflow is set up and the changes are pushed to the repository, the CI/CD pipeline is triggered automatically.
As soon as the push event occurs on the main branch, GitHub Actions picks up the workflow definition from .github/workflows/terraform-gitops-workflow.yml. The workflow runs on an ubuntu-latest runner and executes the Terraform commands step by step.
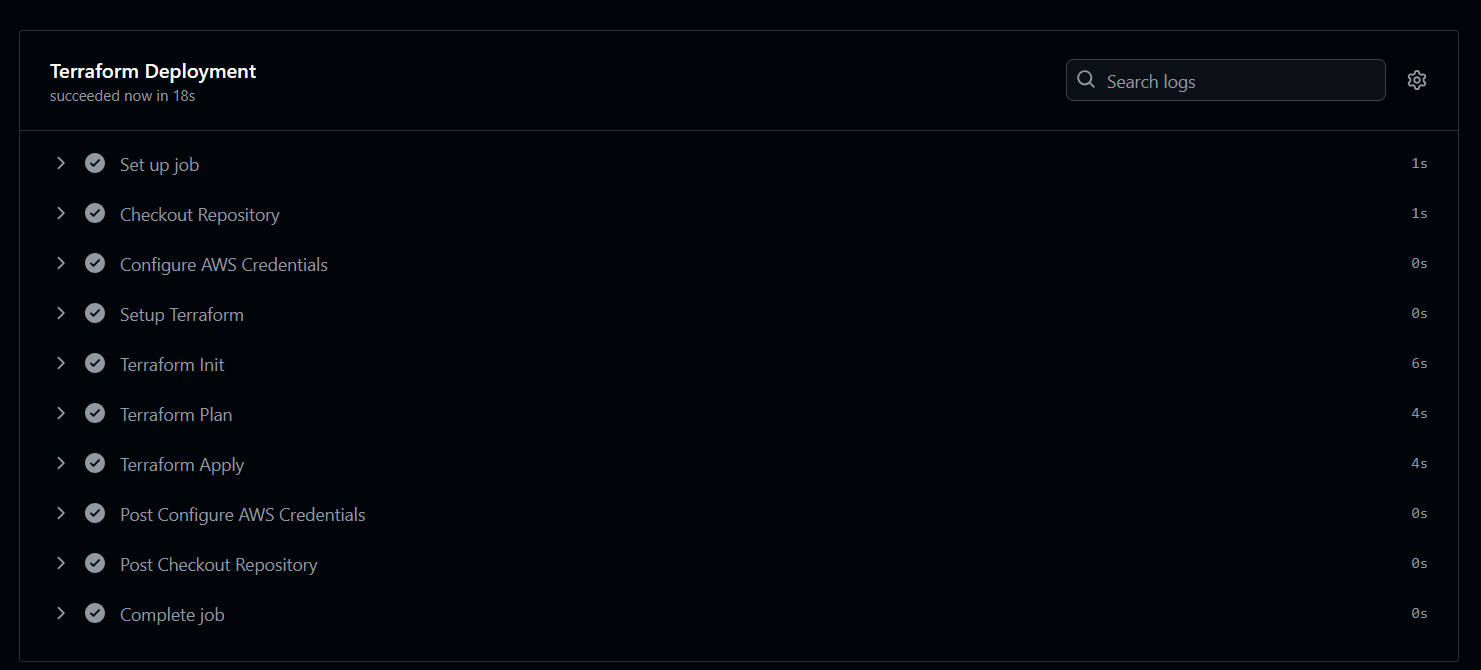
At this point, Terraform is initialized, the plan is generated, and the apply stage successfully provisions the resources. This confirms that GitOps automation is functioning correctly and that changes in the Git repository are directly reflected in the cloud environment.
Best Practices for GitOps with Terraform
Now, to maintain a reliable and secure GitOps workflow with Terraform, several best practices must be followed.
Branch Protection Strategies
One of the first steps is implementing branch protection strategies. Infrastructure changes should never be merged into the main branch without proper review. Enforcing pull request approvals makes sure that every modification is validated by multiple team members before being deployed. This helps catch misconfigurations and potential security risks early in the development cycle.
Granular Permissions
Granular permissions further strengthen security in a GitOps workflow. Not everyone on the team should have permission to approve and apply infrastructure changes. Implementing role-based access control or RBAC makes sure that only authorized users can modify the Terraform state and apply updates to the infra. Restricting write access to the main branch and limiting execution permissions in CI/CD pipelines reduce the risk of accidental or unauthorized deployments.
Monitoring and Alerting
Finally, integrating monitoring and alerting into the workflow improves visibility and response time. Tools like Prometheus, Grafana, and AWS CloudWatch can be used to monitor Terraform deployments, resource utilization, and policy violations. Setting up alerts for failed deployments or drift detections allows teams to respond proactively before issues impact production environments.
Following these best practices ensures that GitOps workflows with Terraform remain secure, efficient, and scalable, minimizing the risks associated with infrastructure automation.
Now, let’s say a DevOps team is provisioning cloud infrastructure using Terraform in a GitOps workflow. A developer makes changes to an S3 bucket policy and an EC2 instance, pushing updates to the Git repository. The Terraform plan runs as part of the CI/CD pipeline, generating an output that must be manually reviewed. The team carefully inspects the Terraform plan logs, checking for misconfigurations, cost impacts, and security risks.
However, this process has limitations:
- Manual Review Overhead – Reviewing Terraform plans line by line is time-consuming, especially for large deployments with multiple resources. The risk of missing misconfigurations is high.
- Lack of Visual Representation – Teams only see text-based plans, making it difficult to understand how resources are connected and what is changing.
- No Automated Policy Enforcement – If a non-compliant change is introduced, such as exposing an EC2 instance to the public internet, it’s up to the reviewers to catch it. If missed, it could lead to security vulnerabilities.
- Cost Uncertainty – Terraform provides no real-time cost analysis. Teams only discover cost implications after deployment, making budget management reactive rather than proactive.
- No Drift Prevention – If an engineer manually modifies an instance outside of GitOps, there is no immediate detection unless a periodic audit or terraform plan run detects it.
This lengthy process means that security gaps, compliance violations, or budget overruns are only caught at later stages, sometimes after deployment.
Firefly to the Rescue
Firefly simplifies and automates this entire process by integrating compliance, cost estimation, and policy enforcement into your GitOps workflows. Instead of relying on manual reviews, Firefly’s workflow feature provides an intuitive graphical interface, where Terraform plans are visualized.
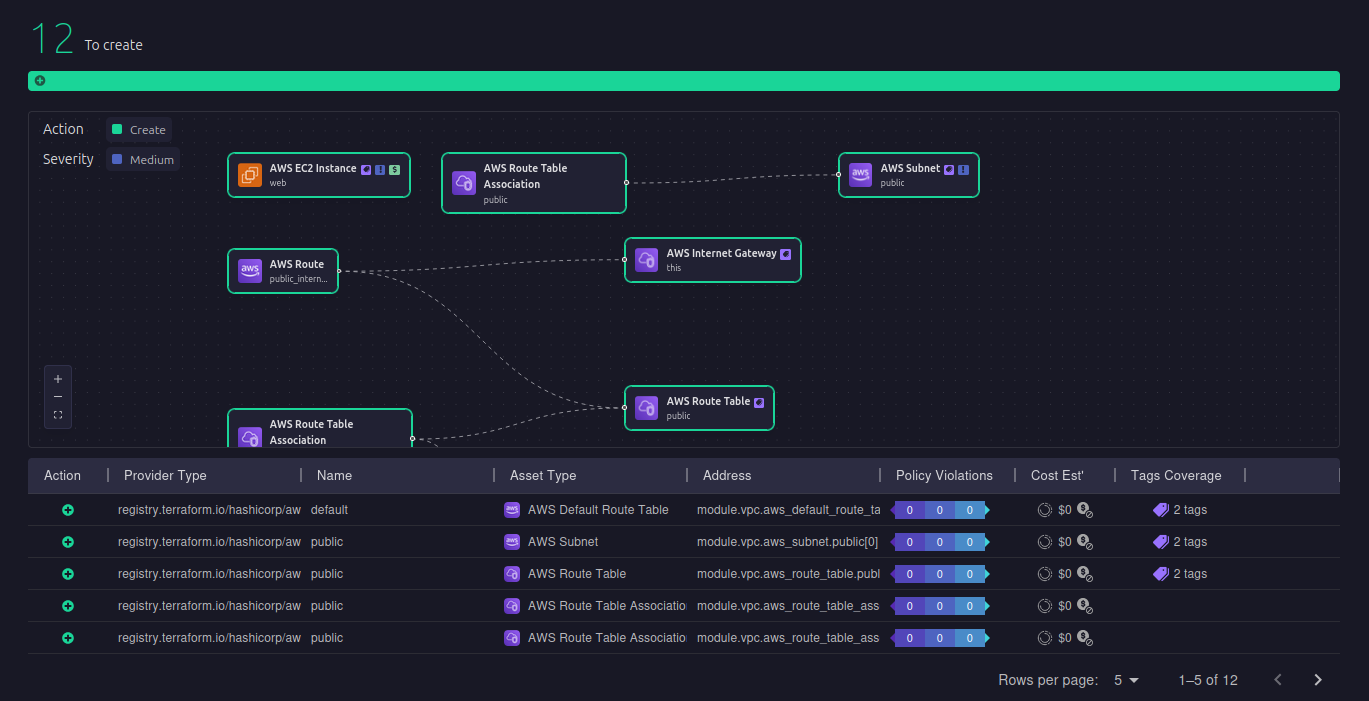
Every planned resource is mapped out, making it easy to see what is being created or modified. Cost estimates are also displayed, ensuring that teams have visibility into financial impacts before deployment.

More importantly, Firefly enforces policies automatically. The screenshot below highlights a Terraform deployment that was blocked due to policy violations. Without Firefly, this would require manual checks, increasing the risk of misconfigurations slipping through.

Here, Firefly immediately flags non-compliant configurations, such as an EC2 instance with a public IP or missing monitoring settings, making sure that they are addressed before deployment. This automated guardrail approach reduces manual intervention while enforcing security best practices.
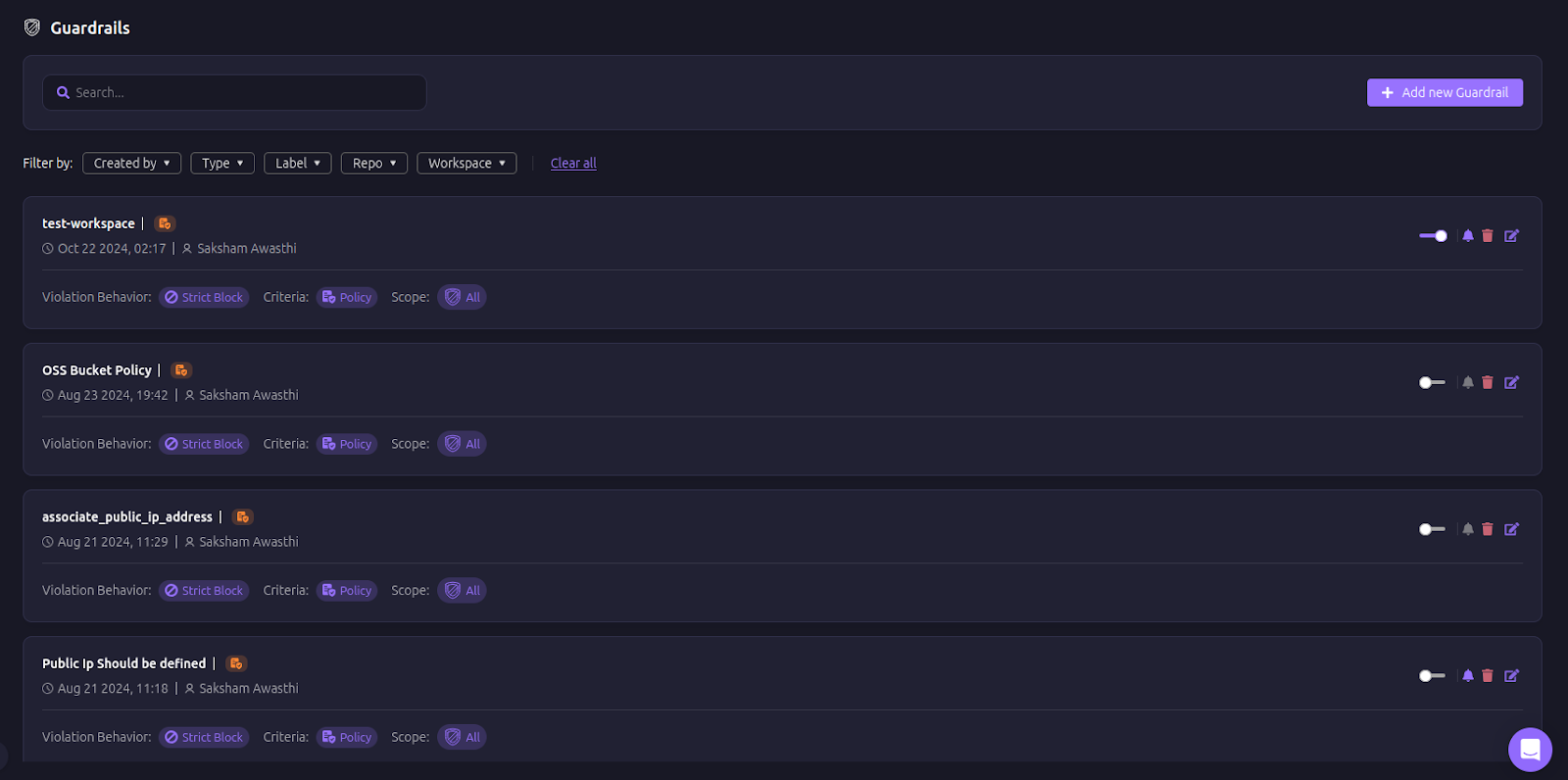
The image above showcases Firefly’s Guardrails, which apply predefined security, compliance, and cost policies across multiple workspaces. Unlike manual Terraform reviews, where every change must be checked individually, Firefly Guardrails enforce organization-wide policies automatically. This ensures consistency across infrastructure deployments and prevents configuration drift by blocking non-compliant changes before they reach production.
With Firefly, teams transition from a reactive, manual approach to an automated, policy-driven workflow. It eliminates tedious Terraform plan reviews, reduces security risks, ensures compliance, and provides real-time cost insights - making GitOps workflows more reliable, efficient, and scalable.
Frequently Asked Question
What is GitOps used for?
GitOps is used to automate and manage infrastructure using version control, ensuring consistency, security, and traceability across cloud deployments.
Is GitHub Actions GitOps?
GitHub Actions is a CI/CD tool that can be used in a GitOps workflow to automate infrastructure deployment, but GitOps itself is a methodology, not a tool.
What is the difference between GitOps and pipeline?
A pipeline automates code deployment, while GitOps extends this by using Git as the single source of truth for infrastructure, ensuring automated, version-controlled infrastructure management.
What are the 3 core practices of GitOps?
GitOps relies on declarative infrastructure, version control as the source of truth, and automated reconciliation to maintain consistency between Git and live infrastructure.